Config
Config provided by player and plugins for developers to customrize player to match their scenario
Required Config
DOM id
- type:
String
id
of a DOM node that you want player to mount on
new Player({
id:'mse'
})
el
- type:
HTMLElement
Another way to tell player the mount node is pass it to config.el
directly
new Player({
el: document.querySelector('#mse'),
})
::: Notice
You need to pass either config.el
or config.id
to player, if both of them exist, player will use the value from config.id
:::
Media Resource URL
- type:
String | Object[]
- default:
''
When the URL is in the form of an array, Source Tag will be used for playback
new Player({
id:'mse',
url: '//abc.com/**/*.mp4',
...
});
// or
new Player({
id:'mse',
url: [
{
src: '//abc.com/**/*.mp4',
type: 'video/mp4'
},
...
],
...
});
Basic config (optional)
width
- type:
Number | String
- default value:
600
Target width of player, use number for a width by px, or string for other units
height
- type:
Number | String
- default value:
337.5
Target height of player, use number for a height by px, or string for other units
autoplay
- type:
Boolean
- default value:
false
If setted with value true
, player would invoke video.play()
after enough media data loaded.
::: Notice In many cases, autoplay action was limited by browser policy, for more details see Known issues about autoplay :::
autoplayMuted
- type:
Boolean
- default value:
false
Autoplay with video muted
videoInit
- type:
Boolean
- default value:
true
Loading media resource immediately after player inited
playsinline
- type:
Boolean
- default value:
true
Enable inline playing mode, would set playsinline DOM attribute to media element. for more details about inline playing mode see new-video-policies-for-ios
WARNING
For devices which's version under ios10, playsinline config doesn't work
defaultPlaybackRate
- type:
Number
- default value:
1
Default playbackrate for media element, reference values: 0.5/0.75/1/1.5/2
volume
- type:
Number
- default value:
0.6
HTMLMediaElement.volume
loop
- type:
Boolean
- default value:
false
HTMLMediaElement.loop
poster
- type:
String
- default value: ``
Poster image url
startTime
- type:
Number
- default value:
0
When setted, player would immediately seek to startTime
after media resource loaded
videoAttributes
- type:
Object
- default value:
{}
DOM attributes for media element, for more details see MediaElement
lang
- type:
String
- default value:
document.documentElement.getAttribute('lang')
||navigator.language
||'zh-cn'
Player language
fluid
- type:
Boolean
- default value:
false
The fluid layout allows the player's width varies to follow the width of the parent element's change, and the height varies according to the height and width proportion of the configuration item (the player's width and height is the internal default value when width and height are not set).
fitVideoSize
- type:
String
- default value:
fixed
fixed
static sizefixWidth
fit by widthfixHeight
fit by height
When video resource was playing, fit raw height、width of video resource to player's
videoFillMode
- type:
String
- default value:
auto
reference values: fillHeight | fillWidth | fill | auto
seekedStatus
- type:
String
- default value:
play
play
pause
auto
Keep player status before seeking
Player status after seeking
progressDot
- type:
Array
- default value:
[]
Marks for progress bar
const progressDot = [{
id: 0,
time: 10, // Shows on progress bar with the position: time / player.duration
text: '01', // Text shows when user hovers on it
duration: 5, // Mark duration
color: 'red', // Mark color
style: {} // Style object for mark DOM
},
...
]
thumbnail
- type:
Object
- default value:
null
Thumbnail for user to preview unplayed video content
var thumbnail = {
urls: [], // Thumbnail picture urls
pic_num: 0, // Total number for all thumbnail pictures
row: 0, // Row number in seperate thumbnail picture
col: 0, // Col number in seperate thumbnail picture
height: 160, // Height for video frame in seperate thumbnail picture by px
width: 90 // Width for video frame in seperate thumbnail picture by px
}
marginControls
- type:
Boolean
- default value:
false
If player render control bar and media element sperately
marginControls:false
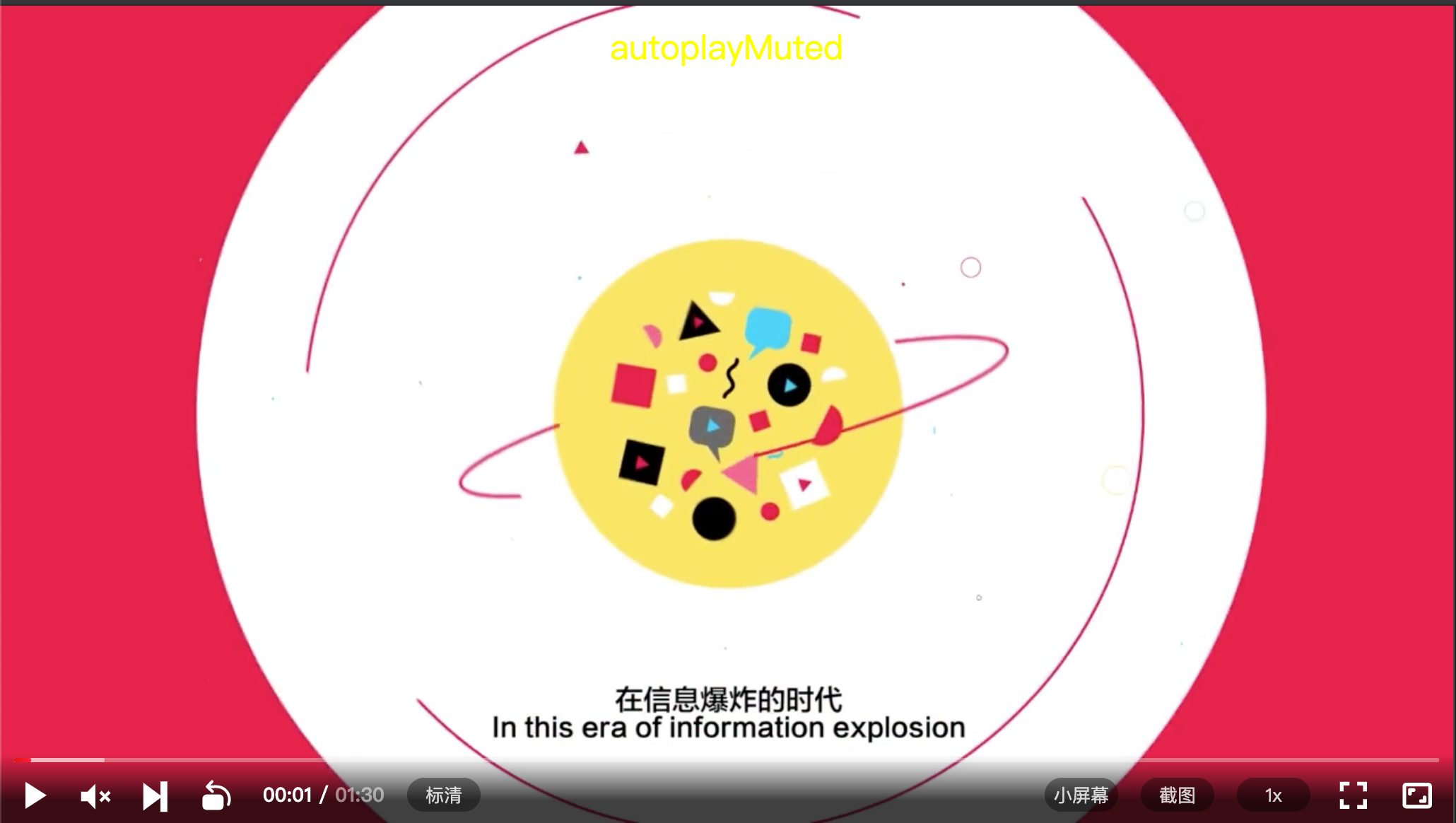
marginControls:true
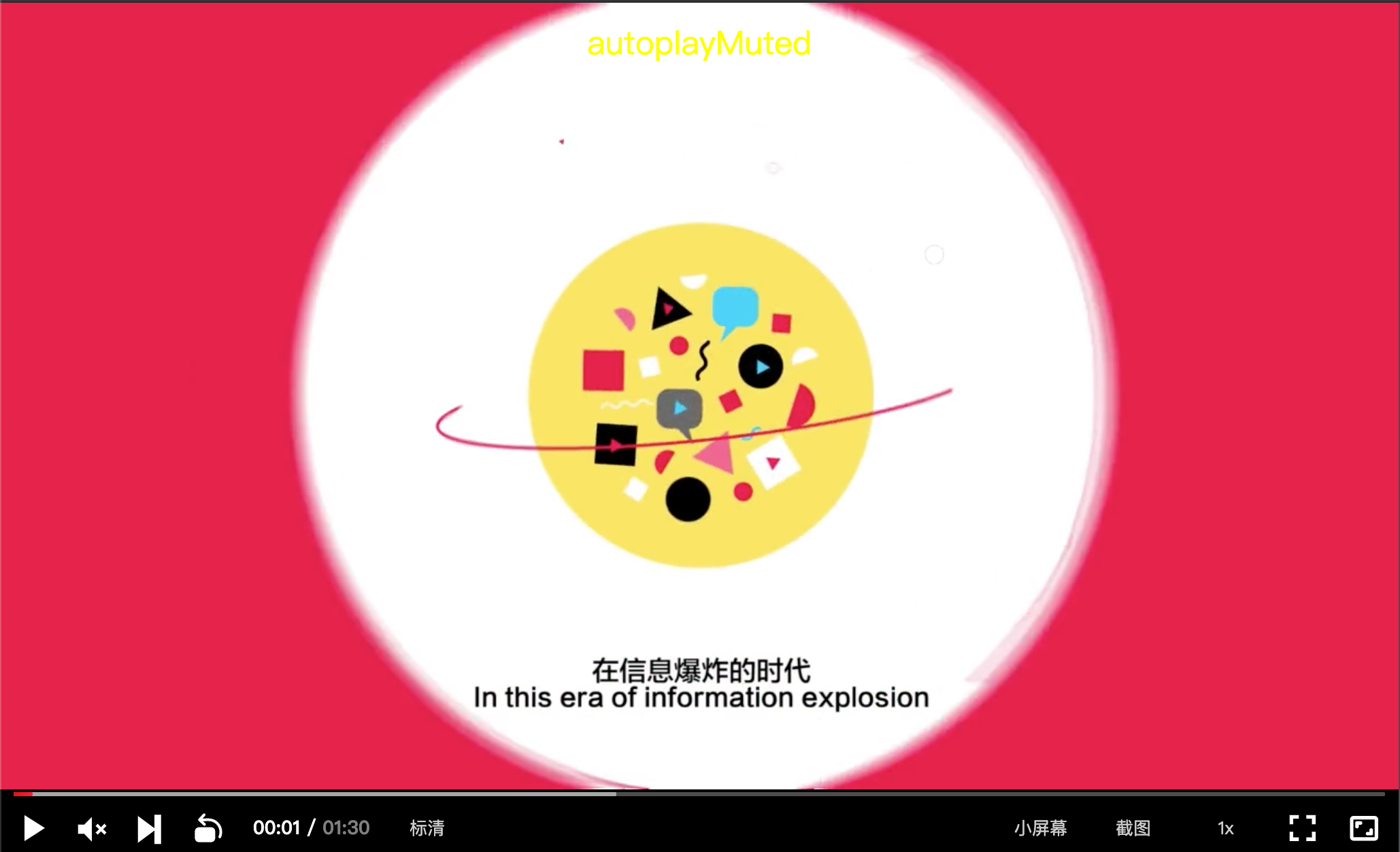
Config for user interaction
closeFocusVideoFocus
- type:
Boolean
- default value:
false
Prevent player from entering focused status when mousemoving on media element Only for PC Browsers
closePauseVideoFocus
- type:
Boolean
- default value:
false
Prevent player from entering focused status when user pause player
closePlayVideoFocus
- type:
Boolean
- default value:
false
Prevent player from entering focused status when user play player
closeVideoClick
- type:
Boolean
- default value:
false
For PC browsers: prevent user from pausing/playing player by click media element For mobile browsers: prevent user pausing/playing player by touch media element
closeVideoDblclick
- type:
Boolean
- default value:
false
For PC browsers: prevent user from pausing/playing player by double click media element For mobile browsers: prevent user pausing/playing player by double touch media element
closeDelayBlur
- type:
Boolean
- default value:
false
Prevent player from auto entering blur status
inactive
- type:
Number
- default value:
3000
Duration after user's last interaction, for player to enter blur status
leavePlayerTime
- type:
Number
- default value:
3000
It only takes effect on the PC side, 'leavePlayerTime'
is the delay time in milliseconds to haide the control bar when the user's mouse leaves the player area. If you want to hide bar immediately, set it to 0
topBarAutoHide
- type:
Boolean
- default value:
true
If auto hide player's top bar (custom plugin's mount position - ROOT_TOP)
enableContextmenu
- type:
Boolean
- default value:
false
Enable context menu for user
allowSeekAfterEnded
- type:
Boolean
- default value:
true
Allow user to perform seek action after video ended
Config for plugins
plugins
type:
array
default value:
[]
Plugin list, use a plugin by pass it to config.plugins. Plugin you passed would be inited by player
import Aplugin from 'Aplugin'
new Player({
el:document.querySelector('#mse'),
url: '{{ video_url }}',
plugins: [Aplugin]
});
You can pass config to a plugin by setting config[pluginName]
// pluginName为'aplugin'
import Aplugin from 'a-xgplayer-plugin'
new Player({
el:document.querySelector('#mse'),
url: '{{ video_url }}',
plugins: [Aplugin],
[pluginName]: {{ pluginConfig }}
});
Lazyload plugin
new Player({
...,
plugins: [{
loader: () => import('a-xgplayer-plugin'), // 异步加载器,需要bundler(webpack、rollup等)支持
timeout: 10000, // Max waiting time for plugin loading
lazy: true, // Mark this plugin as an lazyload plugin
forceBeforeInit: true // Player should waits for plugin loaded before invoking plugin lifecycle callback "beforeInit"
}],
[pluginName]: {{ pluginConfig }}
})
presets
- type:
array
- default value:
[]
Preset list, for more details about preset
, see preset mechanism
ignores
- type:
array
- default value:
[]
Ignore built-in plugins by pluginName
new Player({
...,
ignores: ['start']
})
icons
- type:
object
- default value:
{}
Config for customizing player UI, pass DOMString
、DOM node
、image URL
are all acceptable,
For more details about icons see Icons
import MyPlayIcon from '../icons/my-play-icon.svg';
import MyPauseIcon from '../icons/my-pause-icon.svg';
import Player from 'xgplayer'
const player = new Player({
el:document.querySelector('#mse'),
icons: {
play: MyPlayIcon,
pause: MyPauseIcon
}
})
Notice! if you want to use svg image, please convert it to string before passing. for webpack users we recommand you to use raw-loader
commonStyles
- type:
object
- default value:
{}
Styles config for built-in plugins
{
progressColor: '',
playedColor: '',
// Media cached color
cachedColor: '',
// Progress slider button style
sliderBtnStyle: {},
// Volume bar color
volumeColor: ''
}
controls
- type:
Boolean/object
- default value:
true
Pass false
to disable the control bar, on the other hand you can pass controls config to customize the control bar, for more details see built-in controls plugin
miniprogress
- type:
Boolean
- default value:
false
Pass true
to enable the mini progress bar, which comes out when control bar hide;
screenShot
- type:
Boolean
- default value:
false
Pass true
to enable screenShot, this configuration is the shortcut start configuration of the screenshot plugin, or you can pass in a set of screenshot plugin configuration,for more details see
internalPlugins->screenShot
rotate
- type:
Boolean
- default value:
false
Pass true
to enable rotate, this configuration is the shortcut start configuration of the rotate plugin, or you can pass in a set of rotate plugin configuration,for more details see internalPlugins->rotate
download
- type:
Boolean
- default value:
false
Pass true
to enable download, this configuration is the shortcut start configuration of the download plugin, or you can pass in a set of download plugin configuration,for more details see internalPlugins->download
pip
- type:
Boolean
- default value:
false
Pass true
to enable pip (Picture in Picture), this configuration is the shortcut start configuration of the pip plugin, or you can pass in a set of pip plugin configuration,for more details see internalPlugins->pip
mini
- type:
Boolean
- default value:
false
Pass true
to enable mini, this configuration is the shortcut start configuration of the mini plugin, or you can pass in a set of mini plugin configuration,for more details see internalPlugins->miniscreen
cssFullscreen
- type:
Boolean | {}
- default value:
false
Pass true
to enable cssFullscreen plugin which uses css to realize video full screen, this configuration is the shortcut start configuration of the cssFullscreen plugin, or you can pass in a set of cssFullscreen plugin configuration,for more details see internalPlugins->cssfullscreen
playbackRate
- type:
Boolean | array | {}
- default value:
[0.5, 0.75, 1, 1.5, 2]
Pass false
or array
to control playbackRate plugin, when pass false
, playbackRate plugin will be disabled,for more details see internalPlugins->playbackrate
keyShortcut
- type:
Boolean
- default value:
true
Pass true
to enable keyShortcut, for more details see internalPlugins->keyboard